The topic of this article is the ASP.NET MVC bootstrapping process.
Everything starts in Global.asax:
public class MvcApplication : System.Web.HttpApplication
{
public static void RegisterRoutes(RouteCollection routes)
{
routes.IgnoreRoute("{resource}.axd/{*pathInfo}");
routes.MapRoute(
"Default", // Route name
"{controller}/{action}/{id}", // URL with parameters
new { controller = "Home", action = "Index", id = "" } // Parameter defaults
);
}
protected void Application_Start()
{
RegisterRoutes(RouteTable.Routes);
}
}
This code initializes the ASP.NET Routing framework. The MapRoute() function is an extension method, defined in RouteCollectionExtensions.cs. It’s code is simple:
public static Route MapRoute(this RouteCollection routes, string name, string url, object defaults, object constraints, string[] namespaces) {
if (routes == null) {
throw new ArgumentNullException("routes");
}
if (url == null) {
throw new ArgumentNullException("url");
}
Route route = new Route(url, new MvcRouteHandler()) {
Defaults = new RouteValueDictionary(defaults),
Constraints = new RouteValueDictionary(constraints)
};
if ((namespaces != null) && (namespaces.Length > 0)) {
route.DataTokens = new RouteValueDictionary();
route.DataTokens["Namespaces"] = namespaces;
}
routes.Add(name, route);
return route;
}
Simply creates a route with an MvcRouteHandler at line 9. ASP.NET MVC registers this routing handler to catch the routing requests (MvcRouteHandler.cs):
namespace System.Web.Mvc {
using System.Web.Routing;
public class MvcRouteHandler : IRouteHandler {
protected virtual IHttpHandler GetHttpHandler(RequestContext requestContext) {
return new MvcHandler(requestContext);
}
#region IRouteHandler Members
IHttpHandler IRouteHandler.GetHttpHandler(RequestContext requestContext) {
return GetHttpHandler(requestContext);
}
#endregion
}
}
This code registers the MvcHandler class (MvcHandler.cs) for handling MVC routing requests:
public class MvcHandler : IHttpHandler, IRequiresSessionState
{ [...] }
The class implements the IHttpHandler interface from System.Web.dll to be able to ‘synchronously process HTTP Web requests using custom HTTP handlers’. This interface has only one method:
void ProcessRequest(httpContext context)
The implementation of this method will handle the HTTP request. It builds up the MVC infrastructure and calls the appropriate classes (in transitive way) to select the controller, find the action method and do all the related functionality.
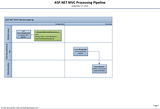
Link: ASP.MET MVC Bootstrapping process